MMC/SD/SDHC card library (2012版)
This project provides a general purpose library which implements read and write support for MMC, SD and SDHC memory cards. It includes
- low-level MMC, SD and SDHC read/write routines
- partition table support
- a simple FAT16/FAT32 read/write implementation
The circuit
The circuit which was mainly used during development consists of an Atmel AVR microcontroller with some passive components. It is quite simple and provides an easy test environment. The circuit which can be downloaded on the project homepage has been improved with regard to operation stability.
I used different microcontrollers during development, the ATmega8 with 8kBytes of flash, and its pin-compatible alternative, the ATmega168 with 16kBytes flash. The first one is the one I started with, but when I implemented FAT16 write support, I ran out of flash space and switched to the ATmega168. For FAT32, an ATmega328 is required.
The circuit board is a self-made and self-soldered board consisting of a single copper layer and standard DIL components, except of the MMC/SD card connector.
The connector is soldered to the bottom side of the board. It has a simple eject button which, when a card is inserted, needs some space beyond the connector itself. As an additional feature the connector has two electrical switches to detect wether a card is inserted and wether this card is write-protected.
Pictures
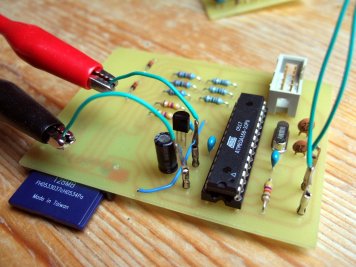
The circuit board used to implement and test this application.
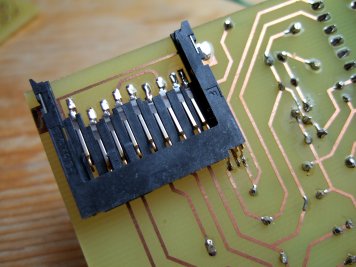
The MMC/SD card connector on the soldering side of the circuit board.
The software
The software is written in C (ISO C99). It might not be the smallest or the fastest one, but I think it is quite flexible. See the project's benchmark page to get an idea of the possible data rates.
I implemented an example application providing a simple command prompt which is accessible via the UART at 9600 Baud. With commands similiar to the Unix shell you can browse different directories, read and write files, create new ones and delete them again. Not all commands are available in all software configurations.
cat <file>
Writes a hexdump of <file> to the terminal.cd <directory>
Changes current working directory to <directory>.disk
Shows card manufacturer, status, filesystem capacity and free storage space.init
Reinitializes and reopens the memory card.ls
Shows the content of the current directory.mkdir <directory>
Creates a directory called <directory>.mv <file> <file_new>
Renames <file> to <file_new>.rm <file>
Deletes <file>.sync
Ensures all buffered data is written to the card.touch <file>
Creates <file>.write <file> <offset>
Writes text to <file>, starting from <offset>. The text is read from the UART, line by line. Finish with an empty line.
The following table shows some typical code sizes in bytes, using the 20090330 release with a buffered read-write MMC/SD configuration, FAT16 and static memory allocation:
layer | code size | static RAM usage |
---|---|---|
MMC/SD | 2410 | 518 |
Partition | 456 | 17 |
FAT16 | 7928 | 188 |
The static RAM is mostly used for buffering memory card access, which improves performance and reduces implementation complexity.
Please note that the numbers above do not include the C library functions used, e.g. some string functions. These will raise the numbers somewhat if they are not already used in other program parts.
When opening a partition, filesystem, file or directory, a little amount of RAM is used, as listed in the following table. Depending on the library configuration, the memory is either allocated statically or dynamically.
descriptor | dynamic/static RAM |
---|---|
partition | 17 |
filesystem | 26 |
file | 53 |
directory | 49 |
Adapting the software to your needs
The only hardware dependent part is the communication layer talking to the memory card. The other parts like partition table and FAT support are completely independent, you could use them even for managing Compact Flash cards or standard ATAPI hard disks.
By changing the MCU* variables in the Makefile, you can use other Atmel microcontrollers or different clock speeds. You might also want to change the configuration defines in the filesfat_config.h, partition_config.h, sd_raw_config.h and sd-reader_config.h. For example, you could disable write support completely if you only need read support.
For further information, visit the project's FAQ page.
Bugs or comments?
If you have comments or found a bug in the software - there might be some of them - you may contact me per mail at feedback@roland-riegel.de.
Acknowledgements
Thanks go to Ulrich Radig, who explained on his homepage how to interface MMC cards to the Atmel microcontroller (http://www.ulrichradig.de/). I adapted his work for my circuit.
Copyright 2006-2012 by Roland Riegel
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License version 2 as published by the Free Software Foundation (http://www.gnu.org/copyleft/gpl.html). At your option, you can alternatively redistribute and/or modify the following files under the terms of the GNU Lesser General Public License version 2.1 as published by the Free Software Foundation (http://www.gnu.org/copyleft/lgpl.html):
- byteordering.c
- byteordering.h
- fat.c
- fat.h
- fat_config.h
- partition.c
- partition.h
- partition_config.h
- sd_raw.c
- sd_raw.h
- sd_raw_config.h
- sd-reader_config.h
MMC/SD/SDHC card library (2010版)
It includes
- low-level MMC, SD and SDHC read/write routines
- partition table support
- a simple FAT16/FAT32 read/write implementation
The circuit
The circuit which was mainly used during development consists of an Atmel AVR microcontroller with some passive components. It is quite simple and provides an easy test environment. The circuit which can be downloaded here has been improved with regard to operation stability.I used different microcontrollers during development, the ATmega8 with 8kBytes of flash, and its pin-compatible alternative, the ATmega168 with 16kBytes flash. The first one is the one I started with, but when I implemented FAT16 write support, I ran out of flash space and switched to the ATmega168. For FAT32, an ATmega328 is required.
The circuit board is a self-made and self-soldered board consisting of a single copper layer and standard DIL components, except of the MMC/SD card connector.
The connector is soldered to the bottom side of the board. It has a simple eject button which, when a card is inserted, needs some space behind the connector itself. As an additional feature the connector has two electrical switches to detect wether a card is inserted and wether this card is write-protected.
Pictures
The circuit board used to implement and test this application.
The MMC/SD card connector on the soldering side of the circuit board.
The software
The software is written in C (ISO C99). It might not be the smallest or the fastest one, but I think it is quite flexible. See the benchmark page to get an idea of the possible data rates.
I implemented an example application providing a simple command prompt which is accessible via the UART at 9600 Baud. With commands similiar to the Unix shell you can browse different directories, read and write files, create new ones and delete them again. Not all commands are available in all software configurations.
layer
code size
static RAM usage
The static RAM is mostly used for buffering memory card access, which improves performance and reduces implementation complexity.
Please note that the numbers above do not include the C library functions used, e.g. some string functions. These will raise the numbers somewhat if they are not already used in other program parts.
When opening a partition, filesystem, file or directory, a little amount of RAM is used, as listed in the following table. Depending on the library configuration, the memory is either allocated statically or dynamically.
descriptor
dynamic/static RAM
By changing the MCU* variables in the Makefile, you can use other Atmel microcontrollers or different clock speeds. You might also want to change the configuration defines in the files fat_config.h, partition_config.h, sd_raw_config.h and sd-reader_config.h. For example, you could disable write support completely if you only need read support.
The software is written in C (ISO C99). It might not be the smallest or the fastest one, but I think it is quite flexible. See the benchmark page to get an idea of the possible data rates.
I implemented an example application providing a simple command prompt which is accessible via the UART at 9600 Baud. With commands similiar to the Unix shell you can browse different directories, read and write files, create new ones and delete them again. Not all commands are available in all software configurations.
- cat <file>
Writes a hexdump of <file> to the terminal. - cd <directory>
Changes current working directory to <directory>. - disk
Shows card manufacturer, status, filesystem capacity and free storage space. - init
Reinitializes and reopens the memory card. - ls
Shows the content of the current directory. - mkdir <directory>
Creates a directory called <directory>. - rm <file>
Deletes <file>. - sync
Ensures all buffered data is written to the card. - touch <file>
Creates <file>. - write <file> <offset>
Writes text to <file>, starting from <offset>. The text is read from the UART, line by line. Finish with an empty line.
layer
code size
static RAM usage
MMC/SD | 2410 | 518 |
Partition | 456 | 17 |
FAT16 | 7928 | 188 |
Please note that the numbers above do not include the C library functions used, e.g. some string functions. These will raise the numbers somewhat if they are not already used in other program parts.
When opening a partition, filesystem, file or directory, a little amount of RAM is used, as listed in the following table. Depending on the library configuration, the memory is either allocated statically or dynamically.
descriptor
dynamic/static RAM
partition | 17 |
filesystem | 26 |
file | 53 |
directory | 49 |
Adapting the software to your needs
The only hardware dependent part is the communication layer talking to the memory card. The other parts like partition table and FAT support are completely independent, you could use them even for managing Compact Flash cards or standard ATAPI hard disks.By changing the MCU* variables in the Makefile, you can use other Atmel microcontrollers or different clock speeds. You might also want to change the configuration defines in the files fat_config.h, partition_config.h, sd_raw_config.h and sd-reader_config.h. For example, you could disable write support completely if you only need read support.
Bugs or comments?
If you have comments or found a bug in the software - there might be some of them - you may contact me per mail at feedback@roland-riegel.de.Acknowledgements
Thanks go to Ulrich Radig, who explained on his homepage how to interface MMC cards to the Atmel microcontroller (http://www.ulrichradig.de/). I adapted his work for my circuit.Copyright © 2006-2010 by Roland Riegel
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License version 2 as published by the Free Software Foundation (http://www.gnu.org/copyleft/gpl.html). At your option, you can alternatively redistribute and/or modify the following files under the terms of the GNU Lesser General Public License version 2.1 as published by the Free Software Foundation (http://www.gnu.org/copyleft/lgpl.html):- byteordering.c
- byteordering.h
- fat.c
- fat.h
- fat16_config.h
- partition.c
- partition.h
- partition_config.h
- sd_raw.c
- sd_raw.h
- sd_raw_config.h
- sd-reader_config.h
Downloads
This section is continuously updated with the newest software revisions. The circuit can be downloaded here and the documentation is available online, but it is also included in the software package. For some frequently asked questions visit the FAQ page. For possible data rates, have a look at the benchmark page.2010-10-10 |
| software sources (285kB) patch since last release (3kB) |
2010-01-10 |
| software sources (284kB) patch since last release (135kB) |
2009-03-30 |
| software sources (273kB) patch since last release (27kB) schematics (211kB) |
2008-11-21 |
| software sources (272kB) patch since last release (121kB) |
2008-06-08 |
| software sources (261kB) patch since last release (53kB) |
2007-12-13 |
| software sources (261kB) patch since last release (67kB) |
2007-06-03 |
| software sources (243kB) patch since last release (11kB) |
2007-03-01 |
| software sources (240kB) patch since last release (6kB) |
2007-01-20 |
| software sources (239kB) patch since last release (9kB) |
2006-11-01 |
| software sources (241kB) patch since last release (50kB) |
2006-09-01 |
| software sources (230kB) patch since last release (7kB) |
2006-08-24 |
| software sources (230kB) patch since last release (19kB) |
2006-08-16 |
| software sources (223kB) patch since last release (16kB) |
2006-08-08 |
| software sources (220kB) patch since last release (41kB) |
2006-03-19 |
| software sources (218kB) patch since last release (8kB) |
2006-03-16 |
| software sources (217kB) schematics (264kB) |
沒有留言:
張貼留言
注意:只有此網誌的成員可以留言。